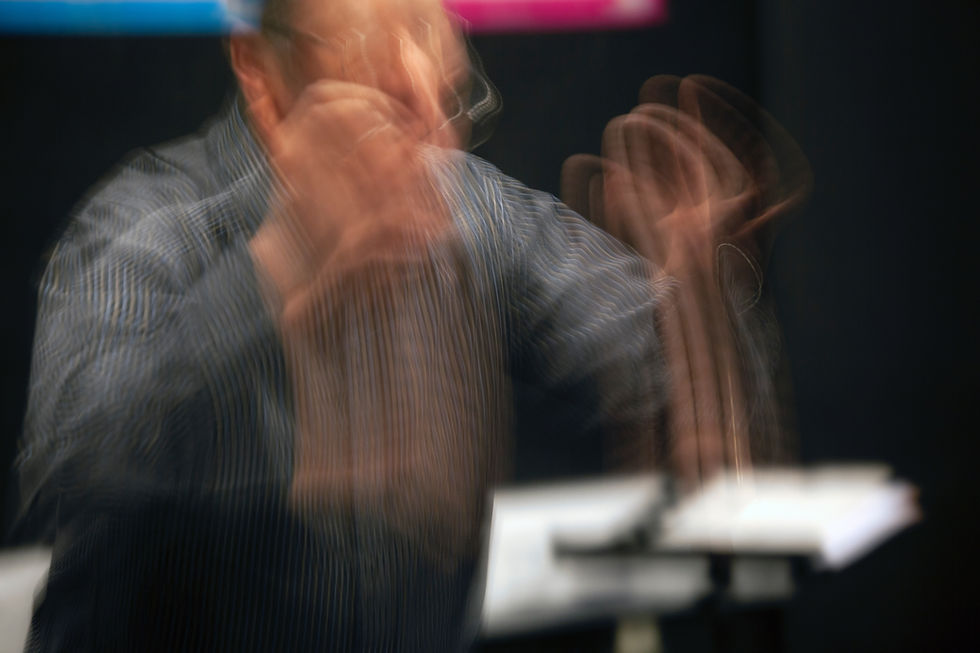
A webhook is a type of API that allows one application (typically a web application) to send data to another application when a particular event takes place. Webhooks are sometimes referred to as “reverse APIs,” because communication is initiated by the application sending the data rather than the one receiving it. They are a way to orchestrate events between multiple tools and the idea can be extended to work in apps, where we can call a function via a deep link, sending a message body in an expected format like json, xml, or yaml.
For example, let's say you have a website that allows users to add a record to a database. When a new record is added, you can use a webhook to send an email notification to a distribution list. This way, that list is notified that the record exists. The record could be a signup for a marketing newsletter, a part that showed up in a warehouse, a security event on a device, or whatever the application does. This follows the old SQL trigger logic, but allows for a more web-oriented approach.
How do Webhooks Work?
Webhooks work by using HTTP requests. When an event occurs, the application triggering the webhook makes an HTTP request to the URL of the application that is receiving the data, or the webhook listener. The HTTP request will include information about the event, such as the date and time of the event, the user who triggered the event, and any other relevant data. That listener can easily be a Lambda hosted in Amazon Web Services or a Google Cloud Function, hosted in Google.
The application that is receiving then processes the HTTP request and takes the appropriate action. For example, if the application is a notification system, it might send an email or a push notification to the user who triggered the event or if it's a devops flow, the receiver might initiate the next step of the build process. Some general benefits to webhooks include:
Real-time data delivery: Webhooks allow developers to deliver data to other applications in real time. This is in contrast to polling, which is a technique where an application periodically requests data from another application. Polling can be inefficient, because it can result in data being delivered late or out of order and there's often overhead to timed polling events, like opening a request for a queue - and logic to derive when and where to run the objects in the queue or time them out.
Increased flexibility: Webhooks give developers and systems administrators more flexibility in how to integrate different applications. With webhooks, developers can choose which events to trigger webhooks, and choose which applications to receive the data. This gives you a lot of control over how your applications interact with each other according to which endpoints are programmed to kick off an external even when an internal event occurs (think of this where a webhook might be synonymous to a public API where our internal actions might be like private APIs).
Reduced development time: Webhooks can help reduce the amount of development time required to integrate different applications. This is because webhooks eliminate the need to write custom code to integrate the applications.
To use webhooks, developers need to:
Find an application that supports webhooks. There are many different applications that support webhooks, so you should be able to find one that meets your needs and for some of us, availability of webhooks now drive the decision for which apps we choose to use.
Configure the webhook in the application that is triggering the event. The configuration process will vary depending on the application, but most give a URL, password, and maybe some field to inject data into the event body.
Configure the webhook in the application that is receiving the data. The configuration process will also vary depending on the application.
When an event occurs in the application that is triggering the event, a webhook will then be sent to the application that is receiving the data.
Example Webhook Listener
Let's use a really generic example and make a Google Cloud Function that listens for a webhook and emails the contents of the webhook to an email address that has been hard coded into the function (note that with environment variables and key sharing and even email services, there are better ways to send an email, but the flow to handle the event.body in the below script should tell the story the listener, which is just a python script, is meant to tell:
function webhook(event, context) {
// Get the email address from the function configuration.
const emailAddress = process.env.EMAIL_ADDRESS;
// Create a new SMTP client.
const smtp = require("smtp");
const client = new smtp();
// Connect to the SMTP server.
client.connect("smtp.gmail.com", 587, {
secure: true,
starttls: true,
auth: {
user: "mygmail@gmail.com",
pass: "my_password",
},
});
// Send the email.
client.sendMail("webhooks@krypted.com", emailAddress, "Webhook notification", event.body);
// Disconnect from the SMTP server.
client.end();
}
To use this function, you would also need to Create a Google Cloud Function, set the EMAIL_ADDRESS environment variable to the email address that you want to send the webhook notifications to, and deploy the function. You'd then have a URL for the function, which is what you put in your script. Should the function be available to any old host in the world, you'd also want to add a password. The system that sends the webhook would then have a URL and password field for sending the event.
Once all that is done (and it could take about 5 minutes before you start testing all the reasons it might fail), the function listens for webhook requests and sends the contents of the requests to the email address that was specified. Many will get a bit more complex and we might internally daisy-chain a bunch of functions that then manipulate the data. There are tools to convert data between formats (e.g. from XML to JSON), lint data structure types, encode/decode, encrypt/decrypt, etc at https://github.com/krypted/tinyconverters to aid in post-processing.
Sending Webhooks
Now let's look at what goes into sending a webhook. Below we'll use a webhookUrl constant, but this would typically be something that could be configured by end users. We'll also just use some generic event data, like if someone created a secret with a type of password in Secret Chest. This would be dynamically generated in the wild but works for our little example TypeScript code:
import http from "http";
const webhookUrl = "https://example.com/webhook";
const data = {
event: "password_created",
eventId: 12345,
secretGuid: alakldsjf24567
customerName: "Chuck U Farley",
customerEmail: "chuckufarley@example.com",
};
const request = new http.Request();
request.method = "POST";
request.headers["Content-Type"] = "application/json";
request.body = JSON.stringify(data);
const response = await http.request(webhookUrl, request);
if (response.status === 200) {
console.log("Webhook sent successfully");
} else {
console.log("Error sending webhook");
}
This script will send a webhook to the URL specified in the webhookUrl variable. The data that is sent to the webhook is specified in the data object. The event property specifies the type of event that is being triggered, the orderId property specifies the ID of the order that was placed, the customerName property specifies the name of the customer who placed the order, and the customerEmail property specifies the email address of the customer who placed the order.
The script will first create a new http.Request object. The method property of the request object is set to POST, which indicates that the request is a POST request. The headers property of the request object is set to an object that contains the Content-Type header. The Content-Type header specifies that the body of the request is JSON. The body property of the request object is set to the JSON-serialized data object.
The script will then use the http.request() method to send the request to the webhook URL. The http.request() method returns a http.Response object. The status property of the response object will be 200 if the webhook was sent successfully. Otherwise, the status property will be a different value.
The script will then check the status property of the response object. If the status property is 200, the script will log a message to the console indicating that the webhook was sent successfully. Otherwise, the script will log a message to the console indicating that an error occurred while sending the webhook.
To tinkerate with the webhooks we've built into Secret Chest (and continue to build), check out our private beta by signing up at www.secretchest.io!
Comments