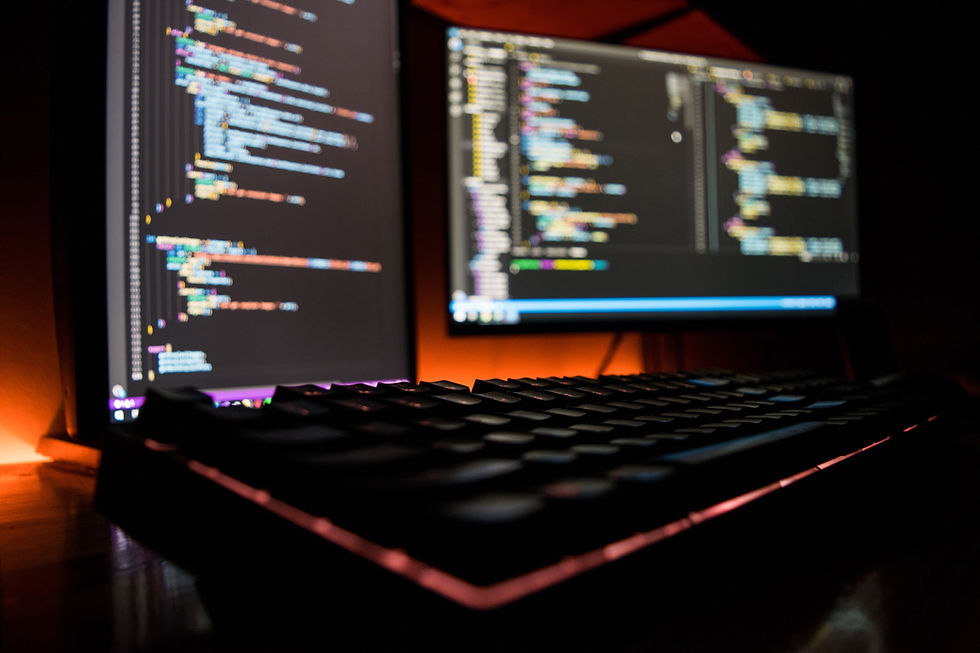
Swift continues to become one of the most efficient languages to build code in for Apple devices. One tool in our developer arsenal is macros, which enable developers to create reusable code snippets for improved maintainability and reduced redundancy.
Swift macros, officially known as "Swift Preprocessor Directives," allow developers to define compile-time code transformations. These directives provide a way to conditionally include or exclude parts of code during the compilation process, offering a level of flexibility and abstraction.
1. Conditional Compilation
Conditional compilation is a primary use case for Swift macros. By employing `#if`, `#elseif`, and `#else` directives, developers can conditionally include or exclude blocks of code based on compilation conditions.
2. Code Generation
Swift macros facilitate code generation, enabling the creation of boilerplate code automatically. This reduces manual effort and ensures consistency in code patterns.
#define LOGGER_METHOD(name) \
func log_##name() { \
print("Logging \(#name)") \
}
LOGGER_METHOD(debug)
LOGGER_METHOD(info)
// Expands to:
// func log_debug() {
// print("Logging debug")
// }
// func log_info() {
// print("Logging info")
// }
3. Version Checking
Swift macros are useful for version-specific code adaptations. Developers can conditionally include code blocks based on the Swift version.
4. Feature Toggles
Implementing feature toggles is simplified with Swift macros. This allows developers to easily enable or disable certain features during development or for specific builds.
Use clear naming conventions when defining macros, use clear and concise names to enhance readability. This makes it easier for other developers to understand the purpose of each macro. Also make sure to provide good documentation to include comments explaining the purpose and usage of macros to aid developers who may encounter the code in the future. Finally, avoid complex logic, so keep macro logic simple to prevent unexpected behavior and improve code reliability. All this helps enhance code organization, reduce redundancy, and adapt code to various conditions.
Comments